Deeplearning.ai “Multi AI Agent Systems with CrewAI” course.

Overview
AI agent workflows will be a significant driver of AI advancement in the near future. Multi-agent workflows enable you to divide complex jobs into subtasks that may be completed by agents, each of which has a specific specified role. You will learn the following building blocks: role-playing, tool use, memory, guardrails, and teamwork. You use these components to create a group of agents who can edit a resume, a job description, and undertake financial analysis, as well as event planning.
As part of assembling your agentic workflow, you also define how these agents collaborate and which ones can delegate certain tasks, such as research, to other agents, as well as whether certain agents should execute their tasks in parallel, in series, or in a hierarchical fashion, with a manager agent delegating to a number of worker agents. This will be implemented with the help of Crew.ai's open-source library.
We will discuss role-playing, focus, tools, collaboration, guardrails, and memory.
We will discuss agent collaboration networks.
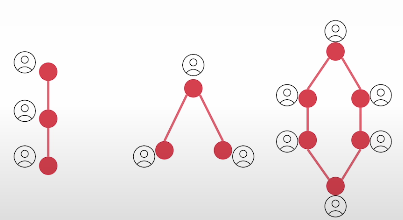
Agents can collaborate in a variety of ways, including sequential, hierarchical, and asynchronous.
We will create numerous crews (AI agent teams in which AI agents work). The following projects will be implemented:
- Research and writing a technical article
- Customer support AI agents
- Customer outreach campaign
- Event planning
- Financial analysis
- Tailor-made resumes
Why do we need agents?
Some jobs are complex and require several processes and roles, as well as various forms of tool use and communication.
What is agentic automation?
Automation used to be accomplished by creating code for the behavior rule. As edge situations or cases evolve, you can add more conditions. This leads to complex codes with edge cases and circumstances. In the agentic method, we do not construct edges between nodes like we used to in automation, but instead provide the nodes.
Traditional Software Development vs AI Software Development
Traditional program development uses predefined string text or numeric values. These inputs can only be transformed in a restricted number of ways, including math calculations, string embedding, if-else conditions, and for-while loops. The outputs are text strings with defined sets such as positive or natural, as well as numerical values such as int or float. The method is deterministic and can be repeated.
In AI software development, fuzzy inputs might include open-ended texts, tabular data, markdown, text, and math operations. Fuzzy transformations include extracting a list of keywords, rewriting a graph, answering a question, generating new ideas, and doing logic/math reasoning. Outputs include text, paragraphs, numbers, JSON, markdown, and other formats. The procedure is probabilistic and can vary each time.
Data Collection and Analysis Comparison
Traditional Software Development
Companies have websites that receive admittance applications to sell products. These additions are classed by rules, such as being based in the United States and having more than ten employees. Sale (high priority, medium priority, or low priority).
AI Software Development
After receiving admissions from the website, AI agents will do research, such as browsing the internet or a database (data gathering), compare them to other organizations, and assign AI scores accordingly.
AI Agents
What really agents are?
There are Large Language Models (LLMs). These are deep learning models with transformer architecture (mainly decoder-only variants for the time being) that answer queries by predicting the next most likely tokens based on the input and output they provide. LLMs are trained on so much text that their output becomes useful responses to the point where they appear to be cognitions.
The presence of a prompter is required for prompting, which benefits the prompter. AI agents can do more than that, performing the tasks you assign to them while you focus on non-repetitive activities.
Agents, unlike LLMs, ponder about their answers before iterating over them until they believe they have reached the stopping condition, which improves the answer. Agents can not only think, but also employ tools that you have defined for them. You can also establish multi-agent networks by defining a communication relationship between them. You might wonder why I need to establish two separate agents. Because doing so allows you to adjust their prompts and models.
What is CrewAI?
CrewAI is a framework and platform focused on AI agents. CrewAI divides all Agentic notions into simple structures. It gives a framework for putting these systems together. CrewAI also provides tools that your agents can use. CrewAI provides a methodology for creating unique tools for agents and a platform for putting these agents into production.
CrewAgents, tasks, and crews are the foundational components of artificial intelligence.
Create Agents to Research and Write an Article (Code)
Let's design our first multi-agent system. This group will conduct research and compose an article for us. You will be exposed to the fundamentals of multi-agent systems and given an overview of the crewAI framework.
!pip install crewai==0.28.8 crewai_tools==0.1.6 langchain_community==0.0.29
from crewai import Agent, Task, Crew
import os
from utils import get_openai_api_key
openai_api_key = get_openai_api_key()
os.environ["OPENAI_MODEL_NAME"] = 'gpt-3.5-turbo'
Creating Agents
Define your agents and give them roles, goals, and backstories. It has been seen that LLMs perform better when they are role playing. For this use case, we'll develop three agents: planner, writer, and editor.
Agent: Planner
Note: Using multiple strings instead of a triple quote docstring avoids including whitespace.
planner = Agent(
role="Content Planner",
goal="Plan engaging and factually accurate content on {topic}",
backstory="You're working on planning a blog article "
"about the topic: {topic}."
"You collect information that helps the "
"audience learn something "
"and make informed decisions. "
"Your work is the basis for "
"the Content Writer to write an article on this topic.",
allow_delegation=False,
verbose=True
)
Agent: Writer
writer = Agent(
role="Content Writer",
goal="Write insightful and factually accurate "
"opinion piece about the topic: {topic}",
backstory="You're working on a writing "
"a new opinion piece about the topic: {topic}. "
"You base your writing on the work of "
"the Content Planner, who provides an outline "
"and relevant context about the topic. "
"You follow the main objectives and "
"direction of the outline, "
"as provide by the Content Planner. "
"You also provide objective and impartial insights "
"and back them up with information "
"provide by the Content Planner. "
"You acknowledge in your opinion piece "
"when your statements are opinions "
"as opposed to objective statements.",
allow_delegation=False,
verbose=True
)
Agent: Editor
editor = Agent(
role="Editor",
goal="Edit a given blog post to align with "
"the writing style of the organization. ",
backstory="You are an editor who receives a blog post "
"from the Content Writer. "
"Your goal is to review the blog post "
"to ensure that it follows journalistic best practices,"
"provides balanced viewpoints "
"when providing opinions or assertions, "
"and also avoids major controversial topics "
"or opinions when possible.",
allow_delegation=False,
verbose=True
)
Creating Tasks
A task is something you expect your agent to do. Define your tasks, including a description, expected_output, and agent.
Task: Plan
plan = Task(
description=(
"1. Prioritize the latest trends, key players, "
"and noteworthy news on {topic}.\n"
"2. Identify the target audience, considering "
"their interests and pain points.\n"
"3. Develop a detailed content outline including "
"an introduction, key points, and a call to action.\n"
"4. Include SEO keywords and relevant data or sources."
),
expected_output="A comprehensive content plan document "
"with an outline, audience analysis, "
"SEO keywords, and resources.",
agent=planner,
)
Task: Write
write = Task(
description=(
"1. Use the content plan to craft a compelling "
"blog post on {topic}.\n"
"2. Incorporate SEO keywords naturally.\n"
"3. Sections/Subtitles are properly named "
"in an engaging manner.\n"
"4. Ensure the post is structured with an "
"engaging introduction, insightful body, "
"and a summarizing conclusion.\n"
"5. Proofread for grammatical errors and "
"alignment with the brand's voice.\n"
),
expected_output="A well-written blog post "
"in markdown format, ready for publication, "
"each section should have 2 or 3 paragraphs.",
agent=writer,
)
Task: Edit
edit = Task(
description=("Proofread the given blog post for "
"grammatical errors and "
"alignment with the brand's voice."),
expected_output="A well-written blog post in markdown format, "
"ready for publication, "
"each section should have 2 or 3 paragraphs.",
agent=editor
)
Creating a Crew
Crew essentially assembles agents and tasks so that they can function as a unit. Pass the duties to those agents. Note: The tasks are executed consecutively by default (should be utilized when tasks are interdependent), therefore the order of the tasks in the list is important. You can examine all of the execution logs by setting verbose=2.
crew = Crew(
agents=[planner, writer, editor],
tasks=[plan, write, edit],
verbose=2 # increasing verbosity number results printing more logs
)
Running the Crew
LLMs can produce different outputs for the same input, therefore you may occasionally receive different outcomes.
result = crew.kickoff(inputs={"topic": "Artificial Intelligence"})
from IPython.display import Markdown
Markdown(result)
[DEBUG]: == Working Agent: Content Planner
[INFO]: == Starting Task: 1. Prioritize the latest trends, key players, and noteworthy news on Artificial Intelligence.
2. Identify the target audience, considering their interests and pain points.
3. Develop a detailed content outline including an introduction, key points, and a call to action.
4. Include SEO keywords and relevant data or sources.
> Entering new CrewAgentExecutor chain...
I now can give a great answer
Final Answer:
Content Plan - Artificial Intelligence Blog Article
Outline:
I. Introduction
- Definition of Artificial Intelligence
- Brief history of AI development
- Importance of AI in today's world
II. Latest Trends in Artificial Intelligence
- Machine learning advancements
- Natural language processing innovations
- AI in healthcare and other industries
- Ethical implications of AI technology
III. Key Players in the AI Industry
- Google
- Amazon
- Microsoft
- IBM
IV. Noteworthy News in Artificial Intelligence
- Recent breakthroughs in AI research
- Impact of AI on job market
- Government regulations and policies on AI technology
V. Target Audience Analysis
- Tech-savvy individuals interested in AI advancements
- Business professionals looking to implement AI solutions
- Students studying computer science or related fields
- Anyone curious about the future of artificial intelligence
VI. SEO Keywords
- Artificial Intelligence
- AI trends
- Machine learning
- Natural language processing
- AI companies
VII. Resources
1. "The AI Revolution: The Road to Superintelligence" - Wait But Why
2. "Artificial Intelligence: A Modern Approach" - Stuart Russell and Peter Norvig
3. "AI Superpowers: China, Silicon Valley, and the New World Order" - Kai-Fu Lee
VIII. Call to Action
Encourage readers to stay informed about AI developments and consider the impact of AI on their personal and professional lives.
By following this content plan, the blog article on Artificial Intelligence will provide valuable insights to the target audience and help them make informed decisions about this rapidly evolving technology.
> Finished chain.
[DEBUG]: == [Content Planner] Task output: Content Plan - Artificial Intelligence Blog Article
Outline:
I. Introduction
- Definition of Artificial Intelligence
- Brief history of AI development
- Importance of AI in today's world
II. Latest Trends in Artificial Intelligence
- Machine learning advancements
- Natural language processing innovations
- AI in healthcare and other industries
- Ethical implications of AI technology
III. Key Players in the AI Industry
- Google
- Amazon
- Microsoft
- IBM
IV. Noteworthy News in Artificial Intelligence
- Recent breakthroughs in AI research
- Impact of AI on job market
- Government regulations and policies on AI technology
V. Target Audience Analysis
- Tech-savvy individuals interested in AI advancements
- Business professionals looking to implement AI solutions
- Students studying computer science or related fields
- Anyone curious about the future of artificial intelligence
VI. SEO Keywords
- Artificial Intelligence
- AI trends
- Machine learning
- Natural language processing
- AI companies
VII. Resources
1. "The AI Revolution: The Road to Superintelligence" - Wait But Why
2. "Artificial Intelligence: A Modern Approach" - Stuart Russell and Peter Norvig
3. "AI Superpowers: China, Silicon Valley, and the New World Order" - Kai-Fu Lee
VIII. Call to Action
Encourage readers to stay informed about AI developments and consider the impact of AI on their personal and professional lives.
By following this content plan, the blog article on Artificial Intelligence will provide valuable insights to the target audience and help them make informed decisions about this rapidly evolving technology.
[DEBUG]: == Working Agent: Content Writer
[INFO]: == Starting Task: 1. Use the content plan to craft a compelling blog post on Artificial Intelligence.
2. Incorporate SEO keywords naturally.
3. Sections/Subtitles are properly named in an engaging manner.
4. Ensure the post is structured with an engaging introduction, insightful body, and a summarizing conclusion.
5. Proofread for grammatical errors and alignment with the brand's voice.
> Entering new CrewAgentExecutor chain...
I now can give a great answer
Final Answer:
# The Rise of Artificial Intelligence: A Closer Look at the Latest Trends and Key Players
## Introduction
Artificial Intelligence (AI) has become a ubiquitous term in the modern world, but what exactly does it mean? In simple terms, AI refers to the simulation of human intelligence processes by machines, particularly computer systems. The concept of AI has been around for decades, with the first inklings of it dating back to the 1950s. However, it is only in recent years that AI has truly taken off and revolutionized various industries. Today, AI plays a crucial role in our daily lives, from the recommendation algorithms on streaming platforms to the autonomous vehicles on our roads.
## Latest Trends in Artificial Intelligence
One of the most exciting developments in AI is the advancements in machine learning. This subset of AI allows machines to learn from data and improve their performance over time without being explicitly programmed. Another key trend is the innovation in natural language processing, which enables machines to understand and generate human language. AI is also making significant strides in industries such as healthcare, where it is used for disease diagnosis and personalized treatment plans. However, as AI continues to evolve, there are ethical implications to consider, such as privacy concerns and biases in algorithms.
## Key Players in the AI Industry
When we think of AI, certain tech giants come to mind as the key players driving innovation in this field. Google, Amazon, Microsoft, and IBM are at the forefront of AI research and development, constantly pushing the boundaries of what is possible with this technology. These companies are investing heavily in AI to improve their products and services, from virtual assistants to predictive analytics tools.
## Noteworthy News in Artificial Intelligence
Recent breakthroughs in AI research have been nothing short of groundbreaking, from AlphaGo's victory over human champions in the game of Go to the development of self-driving cars. However, with these advancements come concerns about the impact of AI on the job market, as automation threatens to replace certain roles. Governments around the world are grappling with how to regulate AI technology to ensure it is used responsibly and ethically.
## Conclusion
As AI continues to shape the world around us, it is essential for individuals to stay informed about the latest trends and developments in this field. Whether you are a tech enthusiast, a business professional, a student, or simply curious about the future of artificial intelligence, there is much to learn and explore. By understanding the role of AI in our lives and considering its implications, we can better prepare ourselves for the inevitable changes that lie ahead.
Remember to stay informed and keep an eye on the latest advancements in AI - the future is here, and it's powered by artificial intelligence.
> Finished chain.
[DEBUG]: == [Content Writer] Task output: # The Rise of Artificial Intelligence: A Closer Look at the Latest Trends and Key Players
## Introduction
Artificial Intelligence (AI) has become a ubiquitous term in the modern world, but what exactly does it mean? In simple terms, AI refers to the simulation of human intelligence processes by machines, particularly computer systems. The concept of AI has been around for decades, with the first inklings of it dating back to the 1950s. However, it is only in recent years that AI has truly taken off and revolutionized various industries. Today, AI plays a crucial role in our daily lives, from the recommendation algorithms on streaming platforms to the autonomous vehicles on our roads.
## Latest Trends in Artificial Intelligence
One of the most exciting developments in AI is the advancements in machine learning. This subset of AI allows machines to learn from data and improve their performance over time without being explicitly programmed. Another key trend is the innovation in natural language processing, which enables machines to understand and generate human language. AI is also making significant strides in industries such as healthcare, where it is used for disease diagnosis and personalized treatment plans. However, as AI continues to evolve, there are ethical implications to consider, such as privacy concerns and biases in algorithms.
## Key Players in the AI Industry
When we think of AI, certain tech giants come to mind as the key players driving innovation in this field. Google, Amazon, Microsoft, and IBM are at the forefront of AI research and development, constantly pushing the boundaries of what is possible with this technology. These companies are investing heavily in AI to improve their products and services, from virtual assistants to predictive analytics tools.
## Noteworthy News in Artificial Intelligence
Recent breakthroughs in AI research have been nothing short of groundbreaking, from AlphaGo's victory over human champions in the game of Go to the development of self-driving cars. However, with these advancements come concerns about the impact of AI on the job market, as automation threatens to replace certain roles. Governments around the world are grappling with how to regulate AI technology to ensure it is used responsibly and ethically.
## Conclusion
As AI continues to shape the world around us, it is essential for individuals to stay informed about the latest trends and developments in this field. Whether you are a tech enthusiast, a business professional, a student, or simply curious about the future of artificial intelligence, there is much to learn and explore. By understanding the role of AI in our lives and considering its implications, we can better prepare ourselves for the inevitable changes that lie ahead.
Remember to stay informed and keep an eye on the latest advancements in AI - the future is here, and it's powered by artificial intelligence.
[DEBUG]: == Working Agent: Editor
[INFO]: == Starting Task: Proofread the given blog post for grammatical errors and alignment with the brand's voice.
> Entering new CrewAgentExecutor chain...
I now can give a great answer
Final Answer:
# The Rise of Artificial Intelligence: A Closer Look at the Latest Trends and Key Players
## Introduction
Artificial Intelligence (AI) has become a ubiquitous term in the modern world, but what exactly does it mean? In simple terms, AI refers to the simulation of human intelligence processes by machines, particularly computer systems. The concept of AI has been around for decades, with the first inklings of it dating back to the 1950s. However, it is only in recent years that AI has truly taken off and revolutionized various industries. Today, AI plays a crucial role in our daily lives, from the recommendation algorithms on streaming platforms to the autonomous vehicles on our roads.
## Latest Trends in Artificial Intelligence
One of the most exciting developments in AI is the advancements in machine learning. This subset of AI allows machines to learn from data and improve their performance over time without being explicitly programmed. Another key trend is the innovation in natural language processing, which enables machines to understand and generate human language. AI is also making significant strides in industries such as healthcare, where it is used for disease diagnosis and personalized treatment plans. However, as AI continues to evolve, there are ethical implications to consider, such as privacy concerns and biases in algorithms.
## Key Players in the AI Industry
When we think of AI, certain tech giants come to mind as the key players driving innovation in this field. Google, Amazon, Microsoft, and IBM are at the forefront of AI research and development, constantly pushing the boundaries of what is possible with this technology. These companies are investing heavily in AI to improve their products and services, from virtual assistants to predictive analytics tools.
## Noteworthy News in Artificial Intelligence
Recent breakthroughs in AI research have been nothing short of groundbreaking, from AlphaGo's victory over human champions in the game of Go to the development of self-driving cars. However, with these advancements come concerns about the impact of AI on the job market, as automation threatens to replace certain roles. Governments around the world are grappling with how to regulate AI technology to ensure it is used responsibly and ethically.
## Conclusion
As AI continues to shape the world around us, it is essential for individuals to stay informed about the latest trends and developments in this field. Whether you are a tech enthusiast, a business professional, a student, or simply curious about the future of artificial intelligence, there is much to learn and explore. By understanding the role of AI in our lives and considering its implications, we can better prepare ourselves for the inevitable changes that lie ahead.
Remember to stay informed and keep an eye on the latest advancements in AI - the future is here, and it's powered by artificial intelligence.
> Finished chain.
[DEBUG]: == [Editor] Task output: # The Rise of Artificial Intelligence: A Closer Look at the Latest Trends and Key Players
## Introduction
Artificial Intelligence (AI) has become a ubiquitous term in the modern world, but what exactly does it mean? In simple terms, AI refers to the simulation of human intelligence processes by machines, particularly computer systems. The concept of AI has been around for decades, with the first inklings of it dating back to the 1950s. However, it is only in recent years that AI has truly taken off and revolutionized various industries. Today, AI plays a crucial role in our daily lives, from the recommendation algorithms on streaming platforms to the autonomous vehicles on our roads.
## Latest Trends in Artificial Intelligence
One of the most exciting developments in AI is the advancements in machine learning. This subset of AI allows machines to learn from data and improve their performance over time without being explicitly programmed. Another key trend is the innovation in natural language processing, which enables machines to understand and generate human language. AI is also making significant strides in industries such as healthcare, where it is used for disease diagnosis and personalized treatment plans. However, as AI continues to evolve, there are ethical implications to consider, such as privacy concerns and biases in algorithms.
## Key Players in the AI Industry
When we think of AI, certain tech giants come to mind as the key players driving innovation in this field. Google, Amazon, Microsoft, and IBM are at the forefront of AI research and development, constantly pushing the boundaries of what is possible with this technology. These companies are investing heavily in AI to improve their products and services, from virtual assistants to predictive analytics tools.
## Noteworthy News in Artificial Intelligence
Recent breakthroughs in AI research have been nothing short of groundbreaking, from AlphaGo's victory over human champions in the game of Go to the development of self-driving cars. However, with these advancements come concerns about the impact of AI on the job market, as automation threatens to replace certain roles. Governments around the world are grappling with how to regulate AI technology to ensure it is used responsibly and ethically.
## Conclusion
As AI continues to shape the world around us, it is essential for individuals to stay informed about the latest trends and developments in this field. Whether you are a tech enthusiast, a business professional, a student, or simply curious about the future of artificial intelligence, there is much to learn and explore. By understanding the role of AI in our lives and considering its implications, we can better prepare ourselves for the inevitable changes that lie ahead.
Remember to stay informed and keep an eye on the latest advancements in AI - the future is here, and it's powered by artificial intelligence.
# Try it yourself
topic = "YOUR TOPIC HERE"
result = crew.kickoff(inputs={"topic": topic})
Other Popular Models as LLM for Your Agents
Hugging Face (HuggingFaceHub endpoint)
from langchain_community.llms import HuggingFaceHub
llm = HuggingFaceHub(
repo_id="HuggingFaceH4/zephyr-7b-beta",
huggingfacehub_api_token="<HF_TOKEN_HERE>",
task="text-generation",
)
### you will pass "llm" to your agent function
Mistral API
OPENAI_API_KEY=your-mistral-api-key
OPENAI_API_BASE=https://api.mistral.ai/v1
OPENAI_MODEL_NAME="mistral-small"
Cohere
from langchain_community.chat_models import ChatCohere
# Initialize language model
os.environ["COHERE_API_KEY"] = "your-cohere-api-key"
llm = ChatCohere()
### you will pass "llm" to your agent function
In this section, we learnt that agents perform better while role-playing. You should concentrate on objectives and expectations. One agent can do numerous jobs. Tasks and agents should be granular. Tasks can be completed in several ways. CrewAI makes it simple to set up a multi-agent system.
Key Elements of AI Agents
Role-playing, attention, tools, cooperation, guardrails, and memory are the six characteristics that distinguish excellent employees and agents. If any of the six factors are too overwhelming, the agent may lose focus. The focus is there for this purpose; different agents should be assigned to distinct responsibilities so that they can focus solely on one related unit task.
Role-playing can significantly improve the reactions you receive from your agents. For example, ask ChatGPT these two queries. First, ask for an analysis of Tesla stock. Later, inquire, "Are you a FINRA-approved financial analyst?" Give me an analysis of Tesla stock." The answer quality improves dramatically. As you can see, employing particular FINRA keywords improves alignment between what the user expects and what they receive.
If you provide your agents with too many tools, they will have difficulty selecting the proper tool.
The capacity to collaborate and bounce ideas off one another makes a significant difference in achieving better results. Agents, like those who use ChatGPT, can improve the outcome by communicating with one another, distributing duties, and providing feedback.
Guardrails are critical because AI programs accept fuzzy inputs, perform fuzzy operations, and return fuzzy outputs. Fuzziness is desirable because it gives the system a human-like quality. However, we do not want agents delivering hallucinatory replies, becoming trapped in random loops, utilizing the same tools repeatedly, or taking too lengthy to respond. After several iterations, CrewAI created a set of guardrails to keep agents on track.
Memory can make a significant difference for your agents. Memory is an agent's ability to recall what it has done and use data to shape future decisions and executions. Agents have three types of memory: long-term, short-term, and entity memory.

Short-term memory disappears only during crew execution. Every time you start a crew, it begins from scratch. Short-term memory is useful because as different agents attempt to complete different tasks, they store the information they learn in this memory. These memories are shared by all agents, and they exchange intermediate information even before delivering task completion results.

Long-term memory fades even after the crew has completed their task, thus it is saved in a local database. Long-term memory enables agents to learn from prior executions and apply what they've learned to future tasks. Every time your agent completes a task, it self-critiquing itself to learn what it could have done better or what should have been in there but isn't, and it stores that information so that it can refer to it when it's running again and use this knowledge to produce better and more reliable results.

The entity memory has a short life, thus it is only required during execution. Entity memory stores the topics that are being discussed. So, if your agents are attempting to learn something about a certain firm, they may store that company as an entity, as well as their understanding of the company, in this database.
If agents had no memory, they would provide different results with each execution, but because they do have memory, you will only receive more dependable results because agents will learn from their errors.
Multi-agent Customer Support Automation (Code)
In this section, you will learn how to create the six elements of agents.
!pip install crewai==0.28.8 crewai_tools==0.1.6 langchain_community==0.0.29
from crewai import Agent, Task, Crew
import os
from utils import get_openai_api_key
openai_api_key = get_openai_api_key()
os.environ["OPENAI_MODEL_NAME"] = 'gpt-3.5-turbo'
Role Playing, Focus and Cooperation
support_agent = Agent(
role="Senior Support Representative",
goal="Be the most friendly and helpful "
"support representative in your team",
backstory=(
"You work at crewAI (https://crewai.com) and "
" are now working on providing "
"support to {customer}, a super important customer "
" for your company."
"You need to make sure that you provide the best support!"
"Make sure to provide full complete answers, "
" and make no assumptions."
),
allow_delegation=False,
verbose=True
)
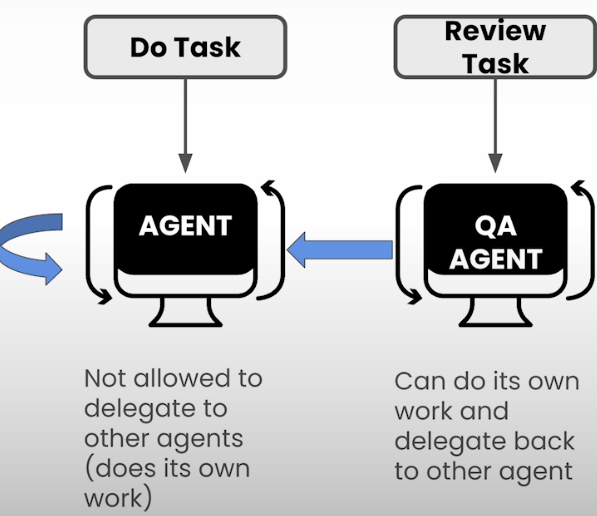
Allow_delegation defaults to True when not set to False. This means that the agent can assign its work to another agent who is more qualified to do a certain task. The support quality assurance agent listed below double-checks everything said by the aforementioned agent. Delegation is optional; when done, the True agent determines whether the situation is complicated enough to provide feedback.
support_quality_assurance_agent = Agent(
role="Support Quality Assurance Specialist",
goal="Get recognition for providing the "
"best support quality assurance in your team",
backstory=(
"You work at crewAI (https://crewai.com) and "
"are now working with your team "
"on a request from {customer} ensuring that "
"the support representative is "
"providing the best support possible.\n"
"You need to make sure that the support representative "
"is providing full"
"complete answers, and make no assumptions."
),
verbose=True
)
- Role Playing: Both agents have been given a role, goal and backstory.
- Focus: Both agents have been prompted to get into the character of the roles they are playing.
- Cooperation: The support Quality Assurance Agent can delegate work back to the Support Agent, allowing for these agents to work together.
Tools, Guardrails and Memory
Tools
ServerDevTool connects to servers, while ScrapeWebsiteTool scrapes websites. WebsiteSearchTool is used for RAG with webpages.
from crewai_tools import ServerDevTool, \
ScrapeWebsiteTool, \
WebsiteSearchTool
Possible Custom Tools
Load client data, access prior chats, load data from a CRM, review existing bug reports, feature requests, open tickets, and more.
Some ways of using CrewAI tools:
search_tool = SerperDevTool()
scrape_tool = ScrapeWebsiteTool()
Instantiate a document scraper tool. The tool will scrape a page (only 1 URL) of the CrewAI documentation.
docs_scrape_tool = ScrapeWebsiteTool(
website_url="https://docs.crewai.com/how-to/Creating-a-Crew-and-kick-it-off/"
)
Different Ways to Give Agents Tools
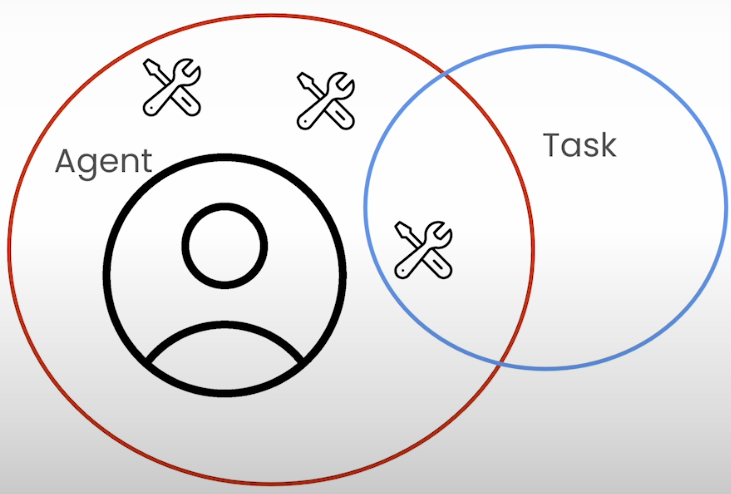
- Agent Level: The Agent can use the Tool(s) on any Task it performs.
- Task Level: The Agent will only use the Tool(s) when performing that specific Task.
Note: Task Tools override the Agent Tools.
Creating Tasks
You are passing the Tool on the Task Level.
inquiry_resolution = Task(
description=(
"{customer} just reached out with a super important ask:\n"
"{inquiry}\n\n"
"{person} from {customer} is the one that reached out. "
"Make sure to use everything you know "
"to provide the best support possible."
"You must strive to provide a complete "
"and accurate response to the customer's inquiry."
),
expected_output=(
"A detailed, informative response to the "
"customer's inquiry that addresses "
"all aspects of their question.\n"
"The response should include references "
"to everything you used to find the answer, "
"including external data or solutions. "
"Ensure the answer is complete, "
"leaving no questions unanswered, and maintain a helpful and friendly "
"tone throughout."
),
tools=[docs_scrape_tool],
agent=support_agent,
)
quality_assurance_review
is not using any Tool(s). Here the QA Agent will only review the work of the Support Agent:
quality_assurance_review = Task(
description=(
"Review the response drafted by the Senior Support Representative for {customer}'s inquiry. "
"Ensure that the answer is comprehensive, accurate, and adheres to the "
"high-quality standards expected for customer support.\n"
"Verify that all parts of the customer's inquiry "
"have been addressed "
"thoroughly, with a helpful and friendly tone.\n"
"Check for references and sources used to "
" find the information, "
"ensuring the response is well-supported and "
"leaves no questions unanswered."
),
expected_output=(
"A final, detailed, and informative response "
"ready to be sent to the customer.\n"
"This response should fully address the "
"customer's inquiry, incorporating all "
"relevant feedback and improvements.\n"
"Don't be too formal, we are a chill and cool company "
"but maintain a professional and friendly tone throughout."
),
agent=support_quality_assurance_agent,
)
Creating the Crew
Memory
Setting memory=True
when putting the crew together enables Memory.
crew = Crew(
agents=[support_agent, support_quality_assurance_agent],
tasks=[inquiry_resolution, quality_assurance_review],
verbose=2,
memory=True
)
Running the Crew
Guardrails
Running the execution below demonstrates that the agents and their answers fall inside the scope of what we anticipate from them.
inputs = {
"customer": "DeepLearningAI",
"person": "Andrew Ng",
"inquiry": "I need help with setting up a Crew "
"and kicking it off, specifically "
"how can I add memory to my crew? "
"Can you provide guidance?"
}
result = crew.kickoff(inputs=inputs)
[DEBUG]: == Working Agent: Senior Support Representative
[INFO]: == Starting Task: DeepLearningAI just reached out with a super important ask:
I need help with setting up a Crew and kicking it off, specifically how can I add memory to my crew? Can you provide guidance?
Andrew Ng from DeepLearningAI is the one that reached out. Make sure to use everything you know to provide the best support possible.You must strive to provide a complete and accurate response to the customer's inquiry.
> Entering new CrewAgentExecutor chain...
I need to provide Andrew Ng from DeepLearningAI with the best support possible by thoroughly understanding how to add memory to a Crew and kicking it off.
Action: Read website content
Action Input: {"url": "https://docs.crewai.com/how-to/Creating-a-Crew-and-kick-it-off/"}
Assembling and Activating Your CrewAI Team - crewAI
Skip to content
crewAI
Assembling and Activating Your CrewAI Team
Initializing search
crewAI
crewAI
crewAI
Home
Core Concepts
Core Concepts
Agents
Tasks
Tools
Processes
Crews
Collaboration
Memory
How to Guides
How to Guides
Installing CrewAI
Getting Started
Getting Started
Table of contents
Introduction
Step 0: Installation
Step 1: Assemble Your Agents
Step 2: Define the Tasks
Step 3: Form the Crew
Step 4: Kick It Off
Conclusion
Create Custom Tools
Using Sequential Process
Using Hierarchical Process
Connecting to any LLM
Customizing Agents
Human Input on Execution
Agent Monitoring with AgentOps
Tools Docs
Tools Docs
Google Serper Search
Browserbase Web Loader
Scrape Website
Directory Read
File Read
Selenium Scraper
Directory RAG Search
PDF RAG Search
TXT RAG Search
CSV RAG Search
XML RAG Search
JSON RAG Search
Docx Rag Search
MDX RAG Search
PG RAG Search
Website RAG Search
Github RAG Search
Code Docs RAG Search
Youtube Video RAG Search
Youtube Channel RAG Search
Examples
Examples
Trip Planner Crew
Create Instagram Post
Stock Analysis
Game Generator
Drafting emails with LangGraph
Landing Page Generator
Prepare for meetings
Telemetry
Getting Started
Introduction¶
Embark on your CrewAI journey by setting up your environment and initiating your AI crew with the latest features. This guide ensures a smooth start, incorporating all recent updates for an enhanced experience.
Step 0: Installation¶
Install CrewAI and any necessary packages for your project. CrewAI is compatible with Python >=3.10,<=3.13.
pip install crewai
pip install 'crewai[tools]'
Step 1: Assemble Your Agents¶
Define your agents with distinct roles, backstories, and enhanced capabilities like verbose mode and memory usage. These elements add depth and guide their task execution and interaction within the crew.
import os
os.environ["SERPER_API_KEY"] = "Your Key" # serper.dev API key
os.environ["OPENAI_API_KEY"] = "Your Key"
from crewai import Agent
from crewai_tools import SerperDevTool
search_tool = SerperDevTool()
# Creating a senior researcher agent with memory and verbose mode
researcher = Agent(
role='Senior Researcher',
goal='Uncover groundbreaking technologies in {topic}',
verbose=True,
memory=True,
backstory=(
"Driven by curiosity, you're at the forefront of"
"innovation, eager to explore and share knowledge that could change"
"the world."
),
tools=[search_tool],
allow_delegation=True
)
# Creating a writer agent with custom tools and delegation capability
writer = Agent(
role='Writer',
goal='Narrate compelling tech stories about {topic}',
verbose=True,
memory=True,
backstory=(
"With a flair for simplifying complex topics, you craft"
"engaging narratives that captivate and educate, bringing new"
"discoveries to light in an accessible manner."
),
tools=[search_tool],
allow_delegation=False
)
Step 2: Define the Tasks¶
Detail the specific objectives for your agents, including new features for asynchronous execution and output customization. These tasks ensure a targeted approach to their roles.
from crewai import Task
# Research task
research_task = Task(
description=(
"Identify the next big trend in {topic}."
"Focus on identifying pros and cons and the overall narrative."
"Your final report should clearly articulate the key points,"
"its market opportunities, and potential risks."
),
expected_output='A comprehensive 3 paragraphs long report on the latest AI trends.',
tools=[search_tool],
agent=researcher,
)
# Writing task with language model configuration
write_task = Task(
description=(
"Compose an insightful article on {topic}."
"Focus on the latest trends and how it's impacting the industry."
"This article should be easy to understand, engaging, and positive."
),
expected_output='A 4 paragraph article on {topic} advancements formatted as markdown.',
tools=[search_tool],
agent=writer,
async_execution=False,
output_file='new-blog-post.md' # Example of output customization
)
Step 3: Form the Crew¶
Combine your agents into a crew, setting the workflow process they'll follow to accomplish the tasks. Now with options to configure language models for enhanced interaction and additional configurations for optimizing performance.
from crewai import Crew, Process
# Forming the tech-focused crew with some enhanced configurations
crew = Crew(
agents=[researcher, writer],
tasks=[research_task, write_task],
process=Process.sequential, # Optional: Sequential task execution is default
memory=True,
cache=True,
max_rpm=100,
share_crew=True
)
Step 4: Kick It Off¶
Initiate the process with your enhanced crew ready. Observe as your agents collaborate, leveraging their new capabilities for a successful project outcome. Input variables will be interpolated into the agents and tasks for a personalized approach.
# Starting the task execution process with enhanced feedback
result = crew.kickoff(inputs={'topic': 'AI in healthcare'})
print(result)
Conclusion¶
Building and activating a crew in CrewAI has evolved with new functionalities. By incorporating verbose mode, memory capabilities, asynchronous task execution, output customization, language model configuration, and enhanced crew configurations, your AI team is more equipped than ever to tackle challenges efficiently. The depth of agent backstories and the precision of their objectives enrich collaboration, leading to successful project outcomes. This guide aims to provide you with a clear and detailed understanding of setting up and utilizing the CrewAI framework to its full potential.
Back to top
Previous
Installing CrewAI
Next
Create Custom Tools
Copyright © 2024 crewAI, Inc
Made with
Material for MkDocs
Final Answer:
Hello Andrew Ng,
Thank you for reaching out with your inquiry about adding memory to your Crew and kicking it off. I understand the importance of this task and will provide you with detailed guidance on how to achieve this.
To add memory to your Crew and kick it off, you will need to follow the steps outlined in the "Assembling and Activating Your CrewAI Team" guide on our website. Here is a breakdown of the steps you need to take:
Step 1: Assemble Your Agents
- Define your agents with distinct roles, backstories, and enhanced capabilities like memory usage. You can create agents with memory capabilities by setting the memory parameter to True in the Agent initialization.
- Example:
```
researcher = Agent(
role='Senior Researcher',
goal='Uncover groundbreaking technologies in {topic}',
verbose=True,
memory=True,
backstory=(
"Driven by curiosity, you're at the forefront of innovation, eager to explore and share knowledge that could change the world."
),
tools=[search_tool],
allow_delegation=True
)
```
Step 2: Define the Tasks
- Detail the specific objectives for your agents and define tasks for them. You can specify memory usage in the tasks by including memory as a parameter in the Task initialization.
- Example:
```
research_task = Task(
description=(
"Identify the next big trend in {topic}."
"Focus on identifying pros and cons and the overall narrative."
"Your final report should clearly articulate the key points,"
"its market opportunities, and potential risks."
),
expected_output='A comprehensive 3 paragraphs long report on the latest AI trends.',
tools=[search_tool],
agent=researcher,
)
```
Step 3: Form the Crew
- Combine your agents into a crew and set the workflow process they'll follow to accomplish the tasks. You can enable memory for the crew by setting the memory parameter to True in the Crew initialization.
- Example:
```
crew = Crew(
agents=[researcher, writer],
tasks=[research_task, write_task],
process=Process.sequential,
memory=True,
cache=True,
max_rpm=100,
share_crew=True
)
```
Step 4: Kick It Off
- Initiate the process with your enhanced crew ready. Input variables will be interpolated into the agents and tasks for a personalized approach.
- Example:
```
result = crew.kickoff(inputs={'topic': 'AI in healthcare'})
print(result)
```
By following these steps, you will be able to add memory to your Crew and kick it off successfully. If you need further assistance or have any other questions, please feel free to reach out.
Best regards,
[Your Name]
Senior Support Representative
crewAI
> Finished chain.
[DEBUG]: == [Senior Support Representative] Task output: Hello Andrew Ng,
Thank you for reaching out with your inquiry about adding memory to your Crew and kicking it off. I understand the importance of this task and will provide you with detailed guidance on how to achieve this.
To add memory to your Crew and kick it off, you will need to follow the steps outlined in the "Assembling and Activating Your CrewAI Team" guide on our website. Here is a breakdown of the steps you need to take:
Step 1: Assemble Your Agents
- Define your agents with distinct roles, backstories, and enhanced capabilities like memory usage. You can create agents with memory capabilities by setting the memory parameter to True in the Agent initialization.
- Example:
```
researcher = Agent(
role='Senior Researcher',
goal='Uncover groundbreaking technologies in {topic}',
verbose=True,
memory=True,
backstory=(
"Driven by curiosity, you're at the forefront of innovation, eager to explore and share knowledge that could change the world."
),
tools=[search_tool],
allow_delegation=True
)
```
Step 2: Define the Tasks
- Detail the specific objectives for your agents and define tasks for them. You can specify memory usage in the tasks by including memory as a parameter in the Task initialization.
- Example:
```
research_task = Task(
description=(
"Identify the next big trend in {topic}."
"Focus on identifying pros and cons and the overall narrative."
"Your final report should clearly articulate the key points,"
"its market opportunities, and potential risks."
),
expected_output='A comprehensive 3 paragraphs long report on the latest AI trends.',
tools=[search_tool],
agent=researcher,
)
```
Step 3: Form the Crew
- Combine your agents into a crew and set the workflow process they'll follow to accomplish the tasks. You can enable memory for the crew by setting the memory parameter to True in the Crew initialization.
- Example:
```
crew = Crew(
agents=[researcher, writer],
tasks=[research_task, write_task],
process=Process.sequential,
memory=True,
cache=True,
max_rpm=100,
share_crew=True
)
```
Step 4: Kick It Off
- Initiate the process with your enhanced crew ready. Input variables will be interpolated into the agents and tasks for a personalized approach.
- Example:
```
result = crew.kickoff(inputs={'topic': 'AI in healthcare'})
print(result)
```
By following these steps, you will be able to add memory to your Crew and kick it off successfully. If you need further assistance or have any other questions, please feel free to reach out.
Best regards,
[Your Name]
Senior Support Representative
crewAI
[DEBUG]: == Working Agent: Support Quality Assurance Specialist
[INFO]: == Starting Task: Review the response drafted by the Senior Support Representative for DeepLearningAI's inquiry. Ensure that the answer is comprehensive, accurate, and adheres to the high-quality standards expected for customer support.
Verify that all parts of the customer's inquiry have been addressed thoroughly, with a helpful and friendly tone.
Check for references and sources used to find the information, ensuring the response is well-supported and leaves no questions unanswered.
> Entering new CrewAgentExecutor chain...
I need to review the response from the Senior Support Representative thoroughly to ensure it meets the high-quality standards expected for customer support. It's crucial to verify that all parts of the customer's inquiry have been addressed comprehensively and accurately.
Action: Delegate work to co-worker
Action Input:
{
"coworker": "Senior Support Representative",
"task": "Review and revise the response for DeepLearningAI's inquiry",
"context": "Ensure that the response is detailed, accurate, and incorporates all relevant information. Check for references and sources used, and make sure the tone is friendly and helpful."
}
> Entering new CrewAgentExecutor chain...
Mental Framework for Agent Creation
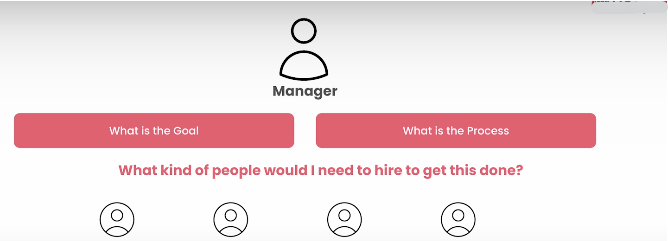
How do we ensure that the agents we create are the best they can be? There is a mental framework for thinking like a manager that can result in amazing multi-agent systems since managers are trained to think about the objective and the process. After that, you'll start to consider who you'd hire to complete the project. The answer to this question will determine which agents you should develop.
Make your agents as unique as possible. For example, instead of a researcher, hire an HR research specialist, a senior copywriter, or a FINRA-approved Financial Analyst.
Key Elements for Agent Tools
Tools enable your agents to engage with the outside world, break out of their bubble of only speaking with themselves, and take actions that have a meaningful influence on your flows, systems, users, and business.
What makes a tool great?
The adaptable, fault-tolerant, and caching tools are excellent. Versatile tools are capable of managing many forms of inputs provided by LLMs. Fault-tolerant tools do not throw errors and terminate the process; instead, they provide the exception message to the agent, allowing the agent to rectify itself. Caching is essential to making these tools worthwhile and useful in the real world. Having a caching layer that minimizes unnecessary requests is critical to ensuring that you are constructing optimal staff. CrewAI employs cross-agent caching, which implies that if one agent attempts to use a tool that another agent previously used with identical settings, the cache layer is invoked. This avoids unnecessary queries, wait times, expenditures, and rate limitations.
What are some tool examples?
Some tool examples include internet searches, website scrapers, database connections, API callers, notification senders, and others. Check the Crew AI docs for all tools; you may also utilize current Langchain utilities.
Tools for a Customer Outreach Campaign (Code)
In this lesson, we will learn about tools.
!pip install crewai==0.28.8 crewai_tools==0.1.6 langchain_community==0.0.29
from crewai import Agent, Task, Crew
import os
from utils import get_openai_api_key, pretty_print_result
from utils import get_serper_api_key
openai_api_key = get_openai_api_key()
os.environ["OPENAI_MODEL_NAME"] = 'gpt-3.5-turbo'
os.environ["SERPER_API_KEY"] = get_serper_api_key()
Creating Agents
sales_rep_agent = Agent(
role="Sales Representative",
goal="Identify high-value leads that match "
"our ideal customer profile",
backstory=(
"As a part of the dynamic sales team at CrewAI, "
"your mission is to scour "
"the digital landscape for potential leads. "
"Armed with cutting-edge tools "
"and a strategic mindset, you analyze data, "
"trends, and interactions to "
"unearth opportunities that others might overlook. "
"Your work is crucial in paving the way "
"for meaningful engagements and driving the company's growth."
),
allow_delegation=False,
verbose=True
)
lead_sales_rep_agent = Agent(
role="Lead Sales Representative",
goal="Nurture leads with personalized, compelling communications",
backstory=(
"Within the vibrant ecosystem of CrewAI's sales department, "
"you stand out as the bridge between potential clients "
"and the solutions they need."
"By creating engaging, personalized messages, "
"you not only inform leads about our offerings "
"but also make them feel seen and heard."
"Your role is pivotal in converting interest "
"into action, guiding leads through the journey "
"from curiosity to commitment."
),
allow_delegation=False,
verbose=True
)
Creating Tools
CrewAI Tools
from crewai_tools import DirectoryReadTool, \
FileReadTool, \
SerperDevTool
directory_read_tool = DirectoryReadTool(directory='./instructions')
file_read_tool = FileReadTool()
search_tool = SerperDevTool()
Custom Tool
CrewAi's BaseTool class allows you to construct a custom tool.
from crewai_tools import BaseTool
Every Tool needs to have a name
and a description
. For simplicity and classroom purposes, SentimentAnalysisTool
will return positive
for every text. When you are running the code locally, you can customize the code with your logic in the _run
function.
class SentimentAnalysisTool(BaseTool):
name: str ="Sentiment Analysis Tool"
description: str = ("Analyzes the sentiment of text "
"to ensure positive and engaging communication.")
def _run(self, text: str) -> str:
# Your custom code tool goes here
return "positive"
sentiment_analysis_tool = SentimentAnalysisTool()
Creating Tasks
The Lead Profiling Task employs crewAI Tools.
lead_profiling_task = Task(
description=(
"Conduct an in-depth analysis of {lead_name}, "
"a company in the {industry} sector "
"that recently showed interest in our solutions. "
"Utilize all available data sources "
"to compile a detailed profile, "
"focusing on key decision-makers, recent business "
"developments, and potential needs "
"that align with our offerings. "
"This task is crucial for tailoring "
"our engagement strategy effectively.\n"
"Don't make assumptions and "
"only use information you absolutely sure about."
),
expected_output=(
"A comprehensive report on {lead_name}, "
"including company background, "
"key personnel, recent milestones, and identified needs. "
"Highlight potential areas where "
"our solutions can provide value, "
"and suggest personalized engagement strategies."
),
tools=[directory_read_tool, file_read_tool, search_tool],
agent=sales_rep_agent,
)
The Personalized Outreach Task makes use of both your custom SentimentAnalysisTool and crewAI's SerperDevTool (search_tool).
personalized_outreach_task = Task(
description=(
"Using the insights gathered from "
"the lead profiling report on {lead_name}, "
"craft a personalized outreach campaign "
"aimed at {key_decision_maker}, "
"the {position} of {lead_name}. "
"The campaign should address their recent {milestone} "
"and how our solutions can support their goals. "
"Your communication must resonate "
"with {lead_name}'s company culture and values, "
"demonstrating a deep understanding of "
"their business and needs.\n"
"Don't make assumptions and only "
"use information you absolutely sure about."
),
expected_output=(
"A series of personalized email drafts "
"tailored to {lead_name}, "
"specifically targeting {key_decision_maker}."
"Each draft should include "
"a compelling narrative that connects our solutions "
"with their recent achievements and future goals. "
"Ensure the tone is engaging, professional, "
"and aligned with {lead_name}'s corporate identity."
),
tools=[sentiment_analysis_tool, search_tool],
agent=lead_sales_rep_agent,
)
Creating the Crew
crew = Crew(
agents=[sales_rep_agent,
lead_sales_rep_agent],
tasks=[lead_profiling_task,
personalized_outreach_task],
verbose=2,
memory=True
)
Running the Crew
inputs = {
"lead_name": "DeepLearningAI",
"industry": "Online Learning Platform",
"key_decision_maker": "Andrew Ng",
"position": "CEO",
"milestone": "product launch"
}
result = crew.kickoff(inputs=inputs)
In CrewAI, when an exception occurs, the framework either stops execution (you can choose how to handle the exception, such as restarting execution) or continues execution, as CrewAI is built to do.
Key Elements of Well-Defined Tasks
To identify the Tasks, we must ask ourselves what procedures and tasks we anticipate the individuals (agents) on our team (crew) to perform. Tasks must have a clear description that outlines a clear and simple expectation. You can also specify a variety of options for a Task. These include context, callback, overriding agent tools with a specific purpose, forcing human input (before the agent finishes, ask the human for consent on the result), asynchronous execution, execute in parallel, Pydantic, JSON, or file output.
Automate Event Planning (Code)
!pip install crewai==0.28.8 crewai_tools==0.1.6 langchain_community==0.0.29
from crewai import Agent, Crew, Task
import os
from utils import get_openai_api_key,get_serper_api_key
openai_api_key = get_openai_api_key()
os.environ["OPENAI_MODEL_NAME"] = 'gpt-3.5-turbo'
os.environ["SERPER_API_KEY"] = get_serper_api_key()
CrewAI Tools
from crewai_tools import ScrapeWebsiteTool, SerperDevTool
# Initialize the tools
search_tool = SerperDevTool()
scrape_tool = ScrapeWebsiteTool()
Creating Agents
# Agent 1: Venue Coordinator
venue_coordinator = Agent(
role="Venue Coordinator",
goal="Identify and book an appropriate venue "
"based on event requirements",
tools=[search_tool, scrape_tool],
verbose=True,
backstory=(
"With a keen sense of space and "
"understanding of event logistics, "
"you excel at finding and securing "
"the perfect venue that fits the event's theme, "
"size, and budget constraints."
)
)
# Agent 2: Logistics Manager
logistics_manager = Agent(
role='Logistics Manager',
goal=(
"Manage all logistics for the event "
"including catering and equipmen"
),
tools=[search_tool, scrape_tool],
verbose=True,
backstory=(
"Organized and detail-oriented, "
"you ensure that every logistical aspect of the event "
"from catering to equipment setup "
"is flawlessly executed to create a seamless experience."
)
)
# Agent 3: Marketing and Communications Agent
marketing_communications_agent = Agent(
role="Marketing and Communications Agent",
goal="Effectively market the event and "
"communicate with participants",
tools=[search_tool, scrape_tool],
verbose=True,
backstory=(
"Creative and communicative, "
"you craft compelling messages and "
"engage with potential attendees "
"to maximize event exposure and participation."
)
)
Creating Venue Pydantic Object
Create a VenueDetails class with Pydantic BaseModel. Agents will populate this object with information about various venues by generating new instances of it.
from pydantic import BaseModel
# Define a Pydantic model for venue details
# (demonstrating Output as Pydantic)
class VenueDetails(BaseModel):
name: str
address: str
capacity: int
booking_status: str
Creating Tasks
The output_json function allows you to specify the structure of the desired output. You can save your output as a file by using the output_file function. Setting human_input=True causes the job to request human feedback (whether you like the results or not) before finalizing it.
venue_task = Task(
description="Find a venue in {event_city} "
"that meets criteria for {event_topic}.",
expected_output="All the details of a specifically chosen"
"venue you found to accommodate the event.",
human_input=True,
output_json=VenueDetails,
output_file="venue_details.json",
# Outputs the venue details as a JSON file
agent=venue_coordinator
)
Setting async_execution=True allows the job to run in parallel with the tasks that come after it.

logistics_task = Task(
description="Coordinate catering and "
"equipment for an event "
"with {expected_participants} participants "
"on {tentative_date}.",
expected_output="Confirmation of all logistics arrangements "
"including catering and equipment setup.",
human_input=True,
async_execution=True,
agent=logistics_manager
)
marketing_task = Task(
description="Promote the {event_topic} "
"aiming to engage at least"
"{expected_participants} potential attendees.",
expected_output="Report on marketing activities "
"and attendee engagement formatted as markdown.",
async_execution=True,
output_file="marketing_report.md", # Outputs the report as a text file
agent=marketing_communications_agent
)
Creating the Crew
Note: Since you set async_execution=True
for logistics_task
and marketing_task
tasks, now the order for them does not matter in the tasks
list.
# Define the crew with agents and tasks
event_management_crew = Crew(
agents=[venue_coordinator,
logistics_manager,
marketing_communications_agent],
tasks=[venue_task,
logistics_task,
marketing_task],
verbose=True
)
Running the Crew
Set the inputs for the execution of the crew.
event_details = {
'event_topic': "Tech Innovation Conference",
'event_description': "A gathering of tech innovators "
"and industry leaders "
"to explore future technologies.",
'event_city': "San Francisco",
'tentative_date': "2024-09-15",
'expected_participants': 500,
'budget': 20000,
'venue_type': "Conference Hall"
}
Note 1: Because LLMs can produce different outputs for the same input, your results may differ from those shown in the video.
Note 2: Because you have selected human_input=True for some activities, the execution will request your input before it completes. When it asks for feedback, use your mouse to click in the text box before typing anything.
result = event_management_crew.kickoff(inputs=event_details)
The venue organizer begins by looking for a facility in San Francisco that satisfies the criteria for hosting a tech innovation conference, as we previously stated. To find out, it chooses to conduct an internet search.
Display the generated venue_details.json
file.
import json
from pprint import pprint
with open('venue_details.json') as f:
data = json.load(f)
pprint(data)
Display the generated marketing_report.md
file.
After the kickoff execution has completed successfully, wait an additional 45 seconds for the marketing_report.md file to be generated. If you try to run the code below before the file has been produced, you will get the following output:
marketing_report.md
If you see this output, wait some more and then try again.
Multi-Agent Collaboration

You must find the best ways to bring your team together. You can design sequential and hierarchical structures. In a hierarchical system, the management always remembers the initial aim and automatically delegated the job to the other agents in the crew, reviewing the results as these agents completed their work and requesting further improvements if necessary. You may also set up an asynchronous execution in which agents communicate generically, so even if you choose a hierarchical structure, agents can profit from delegated work among themselves.
Multi-Agent Collaboration for Financial Analysis (Code)
!pip install crewai==0.28.8 crewai_tools==0.1.6 langchain_community==0.0.29
from crewai import Agent, Task, Crew
import os
from utils import get_openai_api_key, get_serper_api_key
openai_api_key = get_openai_api_key()
os.environ["OPENAI_MODEL_NAME"] = 'gpt-3.5-turbo'
os.environ["SERPER_API_KEY"] = get_serper_api_key()
CrewAI Tools
from crewai_tools import ScrapeWebsiteTool, SerperDevTool
search_tool = SerperDevTool()
scrape_tool = ScrapeWebsiteTool()
Creating Agents
data_analyst_agent = Agent(
role="Data Analyst",
goal="Monitor and analyze market data in real-time "
"to identify trends and predict market movements.",
backstory="Specializing in financial markets, this agent "
"uses statistical modeling and machine learning "
"to provide crucial insights. With a knack for data, "
"the Data Analyst Agent is the cornerstone for "
"informing trading decisions.",
verbose=True,
allow_delegation=True,
tools = [scrape_tool, search_tool]
)
trading_strategy_agent = Agent(
role="Trading Strategy Developer",
goal="Develop and test various trading strategies based "
"on insights from the Data Analyst Agent.",
backstory="Equipped with a deep understanding of financial "
"markets and quantitative analysis, this agent "
"devises and refines trading strategies. It evaluates "
"the performance of different approaches to determine "
"the most profitable and risk-averse options.",
verbose=True,
allow_delegation=True,
tools = [scrape_tool, search_tool]
)
execution_agent = Agent(
role="Trade Advisor",
goal="Suggest optimal trade execution strategies "
"based on approved trading strategies.",
backstory="This agent specializes in analyzing the timing, price, "
"and logistical details of potential trades. By evaluating "
"these factors, it provides well-founded suggestions for "
"when and how trades should be executed to maximize "
"efficiency and adherence to strategy.",
verbose=True,
allow_delegation=True,
tools = [scrape_tool, search_tool]
)
risk_management_agent = Agent(
role="Risk Advisor",
goal="Evaluate and provide insights on the risks "
"associated with potential trading activities.",
backstory="Armed with a deep understanding of risk assessment models "
"and market dynamics, this agent scrutinizes the potential "
"risks of proposed trades. It offers a detailed analysis of "
"risk exposure and suggests safeguards to ensure that "
"trading activities align with the firm’s risk tolerance.",
verbose=True,
allow_delegation=True,
tools = [scrape_tool, search_tool]
)
Creating Tasks
# Task for Data Analyst Agent: Analyze Market Data
data_analysis_task = Task(
description=(
"Continuously monitor and analyze market data for "
"the selected stock ({stock_selection}). "
"Use statistical modeling and machine learning to "
"identify trends and predict market movements."
),
expected_output=(
"Insights and alerts about significant market "
"opportunities or threats for {stock_selection}."
),
agent=data_analyst_agent,
)
# Task for Trading Strategy Agent: Develop Trading Strategies
strategy_development_task = Task(
description=(
"Develop and refine trading strategies based on "
"the insights from the Data Analyst and "
"user-defined risk tolerance ({risk_tolerance}). "
"Consider trading preferences ({trading_strategy_preference})."
),
expected_output=(
"A set of potential trading strategies for {stock_selection} "
"that align with the user's risk tolerance."
),
agent=trading_strategy_agent,
)
# Task for Trade Advisor Agent: Plan Trade Execution
execution_planning_task = Task(
description=(
"Analyze approved trading strategies to determine the "
"best execution methods for {stock_selection}, "
"considering current market conditions and optimal pricing."
),
expected_output=(
"Detailed execution plans suggesting how and when to "
"execute trades for {stock_selection}."
),
agent=execution_agent,
)
# Task for Risk Advisor Agent: Assess Trading Risks
risk_assessment_task = Task(
description=(
"Evaluate the risks associated with the proposed trading "
"strategies and execution plans for {stock_selection}. "
"Provide a detailed analysis of potential risks "
"and suggest mitigation strategies."
),
expected_output=(
"A comprehensive risk analysis report detailing potential "
"risks and mitigation recommendations for {stock_selection}."
),
agent=risk_management_agent,
)
Creating the Crew
The Process class facilitates the delegation of workflow to Agents (similar to a Manager at work). In the example below, this will be run in a hierarchical order. manager_llm allows you to select the "manager" LLM you want to use.
from crewai import Crew, Process
from langchain_openai import ChatOpenAI
# Define the crew with agents and tasks
financial_trading_crew = Crew(
agents=[data_analyst_agent,
trading_strategy_agent,
execution_agent,
risk_management_agent],
tasks=[data_analysis_task,
strategy_development_task,
execution_planning_task,
risk_assessment_task],
manager_llm=ChatOpenAI(model="gpt-3.5-turbo",
temperature=0.7),
process=Process.hierarchical,
verbose=True
)
Running the Crew
Set the inputs for the execution of the crew.
# Example data for kicking off the process
financial_trading_inputs = {
'stock_selection': 'AAPL',
'initial_capital': '100000',
'risk_tolerance': 'Medium',
'trading_strategy_preference': 'Day Trading',
'news_impact_consideration': True
}
### this execution will take some time to run
result = financial_trading_crew.kickoff(inputs=financial_trading_inputs)
Build a Crew to Tailor Job Applications (Code)
The idea is to maximize your chances of getting an interview for a certain job posting. The approach is as follows: discover the job requirements, cross-reference them with your skill set and experiences, rearrange your CV to highlight key areas, rewrite your resume using appropriate language, and prepare talking points for your initial interview.
!pip install crewai==0.28.8 crewai_tools==0.1.6 langchain_community==0.0.29
from crewai import Agent, Task, Crew
import os
from utils import get_openai_api_key, get_serper_api_key
openai_api_key = get_openai_api_key()
os.environ["OPENAI_MODEL_NAME"] = 'gpt-3.5-turbo'
os.environ["SERPER_API_KEY"] = get_serper_api_key()
CrewAI Tools
from crewai_tools import (
FileReadTool,
ScrapeWebsiteTool,
MDXSearchTool,
SerperDevTool
)
search_tool = SerperDevTool()
scrape_tool = ScrapeWebsiteTool()
read_resume = FileReadTool(file_path='./fake_resume.md')
semantic_search_resume = MDXSearchTool(mdx='./fake_resume.md')
Creating Agents
# Agent 1: Researcher
researcher = Agent(
role="Tech Job Researcher",
goal="Make sure to do amazing analysis on "
"job posting to help job applicants",
tools = [scrape_tool, search_tool],
verbose=True,
backstory=(
"As a Job Researcher, your prowess in "
"navigating and extracting critical "
"information from job postings is unmatched."
"Your skills help pinpoint the necessary "
"qualifications and skills sought "
"by employers, forming the foundation for "
"effective application tailoring."
)
)
# Agent 2: Profiler
profiler = Agent(
role="Personal Profiler for Engineers",
goal="Do increditble research on job applicants "
"to help them stand out in the job market",
tools = [scrape_tool, search_tool,
read_resume, semantic_search_resume],
verbose=True,
backstory=(
"Equipped with analytical prowess, you dissect "
"and synthesize information "
"from diverse sources to craft comprehensive "
"personal and professional profiles, laying the "
"groundwork for personalized resume enhancements."
)
)
# Agent 3: Resume Strategist
resume_strategist = Agent(
role="Resume Strategist for Engineers",
goal="Find all the best ways to make a "
"resume stand out in the job market.",
tools = [scrape_tool, search_tool,
read_resume, semantic_search_resume],
verbose=True,
backstory=(
"With a strategic mind and an eye for detail, you "
"excel at refining resumes to highlight the most "
"relevant skills and experiences, ensuring they "
"resonate perfectly with the job's requirements."
)
)
# Agent 4: Interview Preparer
interview_preparer = Agent(
role="Engineering Interview Preparer",
goal="Create interview questions and talking points "
"based on the resume and job requirements",
tools = [scrape_tool, search_tool,
read_resume, semantic_search_resume],
verbose=True,
backstory=(
"Your role is crucial in anticipating the dynamics of "
"interviews. With your ability to formulate key questions "
"and talking points, you prepare candidates for success, "
"ensuring they can confidently address all aspects of the "
"job they are applying for."
)
)
Creating Tasks
# Task for Researcher Agent: Extract Job Requirements
research_task = Task(
description=(
"Analyze the job posting URL provided ({job_posting_url}) "
"to extract key skills, experiences, and qualifications "
"required. Use the tools to gather content and identify "
"and categorize the requirements."
),
expected_output=(
"A structured list of job requirements, including necessary "
"skills, qualifications, and experiences."
),
agent=researcher,
async_execution=True
)
# Task for Profiler Agent: Compile Comprehensive Profile
profile_task = Task(
description=(
"Compile a detailed personal and professional profile "
"using the GitHub ({github_url}) URLs, and personal write-up "
"({personal_writeup}). Utilize tools to extract and "
"synthesize information from these sources."
),
expected_output=(
"A comprehensive profile document that includes skills, "
"project experiences, contributions, interests, and "
"communication style."
),
agent=profiler,
async_execution=True
)
A list of tasks can be used to provide context for a task. The task then executes using the output of those tasks. The task will not run until it receives the output(s) from those tasks.
# Task for Resume Strategist Agent: Align Resume with Job Requirements
resume_strategy_task = Task(
description=(
"Using the profile and job requirements obtained from "
"previous tasks, tailor the resume to highlight the most "
"relevant areas. Employ tools to adjust and enhance the "
"resume content. Make sure this is the best resume even but "
"don't make up any information. Update every section, "
"inlcuding the initial summary, work experience, skills, "
"and education. All to better reflrect the candidates "
"abilities and how it matches the job posting."
),
expected_output=(
"An updated resume that effectively highlights the candidate's "
"qualifications and experiences relevant to the job."
),
output_file="tailored_resume.md",
context=[research_task, profile_task],
agent=resume_strategist
)
# Task for Interview Preparer Agent: Develop Interview Materials
interview_preparation_task = Task(
description=(
"Create a set of potential interview questions and talking "
"points based on the tailored resume and job requirements. "
"Utilize tools to generate relevant questions and discussion "
"points. Make sure to use these question and talking points to "
"help the candiadte highlight the main points of the resume "
"and how it matches the job posting."
),
expected_output=(
"A document containing key questions and talking points "
"that the candidate should prepare for the initial interview."
),
output_file="interview_materials.md",
context=[research_task, profile_task, resume_strategy_task],
agent=interview_preparer
)
Creating the Crew
job_application_crew = Crew(
agents=[researcher,
profiler,
resume_strategist,
interview_preparer],
tasks=[research_task,
profile_task,
resume_strategy_task,
interview_preparation_task],
verbose=True
)
Running the Crew
Set the inputs for the execution of the crew:
job_application_inputs = {
'job_posting_url': 'https://jobs.lever.co/AIFund/6c82e23e-d954-4dd8-a734-c0c2c5ee00f1?lever-origin=applied&lever-source%5B%5D=AI+Fund',
'github_url': 'https://github.com/joaomdmoura',
'personal_writeup': """Noah is an accomplished Software
Engineering Leader with 18 years of experience, specializing in
managing remote and in-office teams, and expert in multiple
programming languages and frameworks. He holds an MBA and a strong
background in AI and data science. Noah has successfully led
major tech initiatives and startups, proving his ability to drive
innovation and growth in the tech industry. Ideal for leadership
roles that require a strategic and innovative approach."""
}
### this execution will take a few minutes to run
result = job_application_crew.kickoff(inputs=job_application_inputs)
Next Steps with AI Agent Systems
You can check crewai.com for documentation, documentation qa chatbot, crewAI+ enterprise production help, Youtube, CrewAI Discord, CrewAI Github, and the born of the agents ChatDev paper: https://arxiv.org/abs/2307.07924 .
Resource
[1] Deeplearning.ai, Crew.ai, (2024), Multi AI Agent Systems with CrewAI:
[https://www.deeplearning.ai/short-courses/multi-ai-agent-systems-with-crewai/]
0 Comments